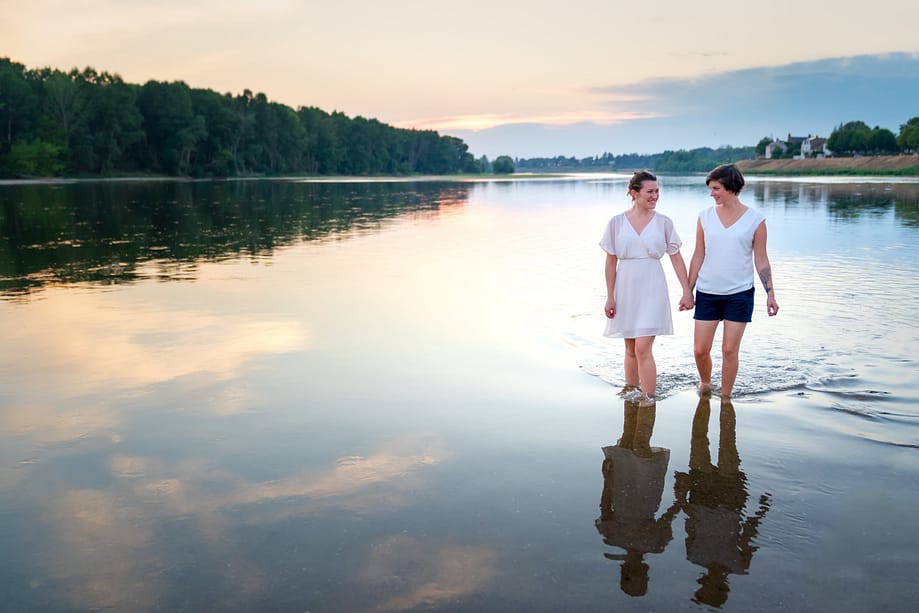
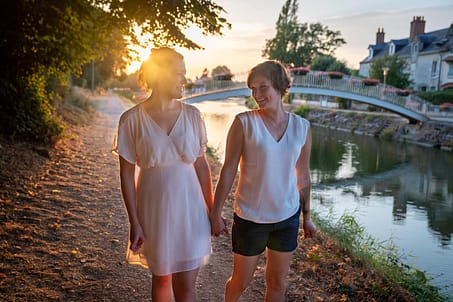














”
Nous avons profité de la superbe lumière d'une belle soirée de juillet pour réaliser cette superbe séance d'engagement quelques semaines avant qu'elles ne se marient en Août 2020.
Un grand moment de complicité, de tendresse et d'Amour !
C'est dans des moments comme celui-ci que je mesure l'immense bonheur d'exercer mon métier de photographe...
- yannick le bricquir
Magnifiques photos.
Merci beaucoup !